What is cross-site scripting (XSS)?
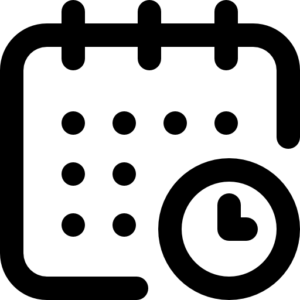
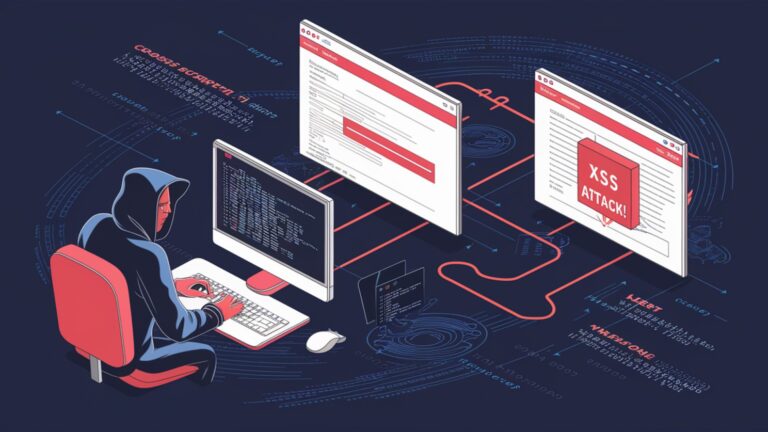
Cross-site scripting, also known as XSS, occurs when a hacker injects a script into a website or application. Hackers often send users a link and convince them to click it. If the website or application doesn’t validate the data, a malicious link can cause the hacker’s code to run on the user’s device. This allows the hacker to steal the user’s session cookies.
How XSS Attacks Work
Identifying vulnerable input fields: The attacker finds a web application that accepts user input and reflects it to the user without proper validation or encoding. Common vulnerable inputs include search boxes, comment fields, or URL parameters.
Injecting malicious script: An attacker creates a malicious script (such as JavaScript) and injects it into a vulnerable input field. This can be done through form submission, URL manipulation, or another input method.
Execution in the user’s browser: When a user visits a compromised website, the injected script is served as part of the page content. The user’s browser will execute the script because it will assume that it is part of a legitimate website.
Example:-
A simple example of a reflected XSS attack might look like this:
- The attacker crafts a URL that includes a malicious script in a parameter:
http://example.com/search?q=<script>alert(‘XSS’)</script>
- When a user clicks on this URL, the web application reflects the parameter q back to the web page without proper sanitization:
<html>
<body>
<h1>Search Results</h1>
<p>You searched for: <script>alert(‘XSS’)</script></p>
</body>
</html>
- The user’s browser executes the script within the context of the web page, triggering an alert box with the message “XSS”
Common Vulnerable Areas for Cross-Site Scripting
Input Fields:
- Search Boxes: Attackers can inject scripts into search queries that are reflected in the search results.
- Comment Sections: Scripts can be embedded in user comments and displayed when the comment is rendered.
- Form Fields: Fields like login forms, contact forms, and feedback forms can be used to inject scripts.
URL Parameters:
- Query Strings: Parameters in the URL that are not properly sanitized can be manipulated to include malicious scripts.
- Path Parameters: URLs that include user-generated content in the path can be exploited if not properly handled.
Third-Party Widgets and Plugins:
- Advertisements: Malicious ads can inject scripts into the host web page.
- Social Media Widgets: Plugins for comments, likes, and shares can be vectors for XSS if not securely implemented.
API Endpoints:
- RESTful APIs: Endpoints that reflect user input in responses can be exploited for XSS.
- GraphQL APIs: Queries that include unsanitized user input can be a target for XSS.
Detection and Prevention of Cross-Site Scripting (XSS)
Detection
1. Automated Scanners:
- OWASP ZAP: An open-source tool for finding security vulnerabilities in web applications, including XSS.
- Burp Suite: A comprehensive web vulnerability scanner that can detect XSS vulnerabilities.
2. Manual Testing:
- Input Testing: Manually test input fields, URL parameters, and other user input points by injecting typical XSS payloads such as <script>alert(‘XSS’)</script
- Browser Developer Tools: Use tools like Chrome DevTools to inspect and manipulate DOM elements to check for XSS vulnerabilities.
3. Code Reviews:
- Static Analysis: Use static code analysis tools to detect potential XSS vulnerabilities in your source code.
- Manual Code Review: Manually review your code to identify places where user input is not being handled correctly.
4. Content Security Policy (CSP)
- Define CSP Headers: Use Content Security Policy headers to restrict the sources from which scripts can be loaded and executed.
Basic Example: Vulnerable to XSS
This example shows a simple PHP script that takes user input and displays it without any sanitization, making it vulnerable to XSS attacks
<?php
if ($_SERVER[‘REQUEST_METHOD’] === ‘POST’) {
$userInput = $_POST[‘input’];
// Vulnerable to XSS
echo “User Input: ” . $userInput;
}
?>
<!DOCTYPE html>
<html>
<head>
<title>XSS Vulnerability Example</title>
</head>
<body>
<h1>XSS Vulnerability Example</h1>
<form method=”post” action=””>
<label for=”input”>Enter text:</label>
<input type=”text” id=”input” name=”input”>
<button type=”submit”>Submit</button>
</form>
</body>
</html>
Sanitizing User Input with htmlspecialchars
<?php
if ($_SERVER[‘REQUEST_METHOD’] === ‘POST’) {
$userInput = $_POST[‘input’];
// Sanitizing user input
$sanitizedInput = htmlspecialchars($userInput, ENT_QUOTES, ‘UTF-8’);
echo “Sanitized Input: ” . $sanitizedInput;
}
?>
<!DOCTYPE html>
<html>
<head>
<title>XSS Prevention Example</title>
</head>
<body>
<h1>XSS Prevention Example</h1>
<form method=”post” action=””>
<label for=”input”>Enter text:</label>
<input type=”text” id=”input” name=”input”>
<button type=”submit”>Submit</button>
</form>
</body>
</html>
Explanation
- Vulnerable Example:- This code accepts user input from a form and returns it directly to the user without sanitizing it, making it vulnerable to XSS attacks.
- Sanitizing with htmlspecialchars:-The htmlspecialchars function converts special characters to HTML entities to prevent the browser from interpreting them as HTML or JavaScript. This is a simple yet effective method of basic XSS prevention.
Impact of an XSS Attack
E-commerce Website:
Scenario: An attacker exploits an XSS vulnerability on a popular e-commerce site.
Impact: The attacker can steal users’ session cookies, allowing them to hijack accounts. They could make unauthorized purchases or access users’ personal and payment information.
Social Media Platform:
Scenario: An XSS vulnerability in a social media platform is exploited.
Impact: The attacker can post malicious content or links that spread the XSS attack to other users. They can steal personal messages and user data, damaging user trust and platform integrity.
Online Banking Application:
Scenario: An XSS vulnerability in an online banking portal.
Impact: The attacker can perform session hijacking, leading to unauthorized transactions and potential financial loss for the users. This can have severe consequences, including legal action and loss of reputation for the bank.
Healthcare Application:
Scenario: An XSS attack on a healthcare management system.
Impact: The attacker can access sensitive patient data, such as medical records and personal information. This breach can lead to privacy violations and legal consequences for the healthcare provider.
Conclusion
Cross-site scripting (XSS) poses a significant risk to web application security when a malicious attacker injects malicious script into web pages visited by other users. To protect against XSS, it is important to sanitize user input and implement output encoding. Additionally, security libraries and Content Security Policy (CSP) can provide additional layers of defense. To maintain the security of your web applications, it is important to perform regular vulnerability assessments. Following these measures will help prevent XSS attacks and ensure a safe browsing experience for users. At IOSCAPE we are committed to improving web security and protecting critical data of our customers from XSS Attacks and other threats to ensure a safer digital environment.